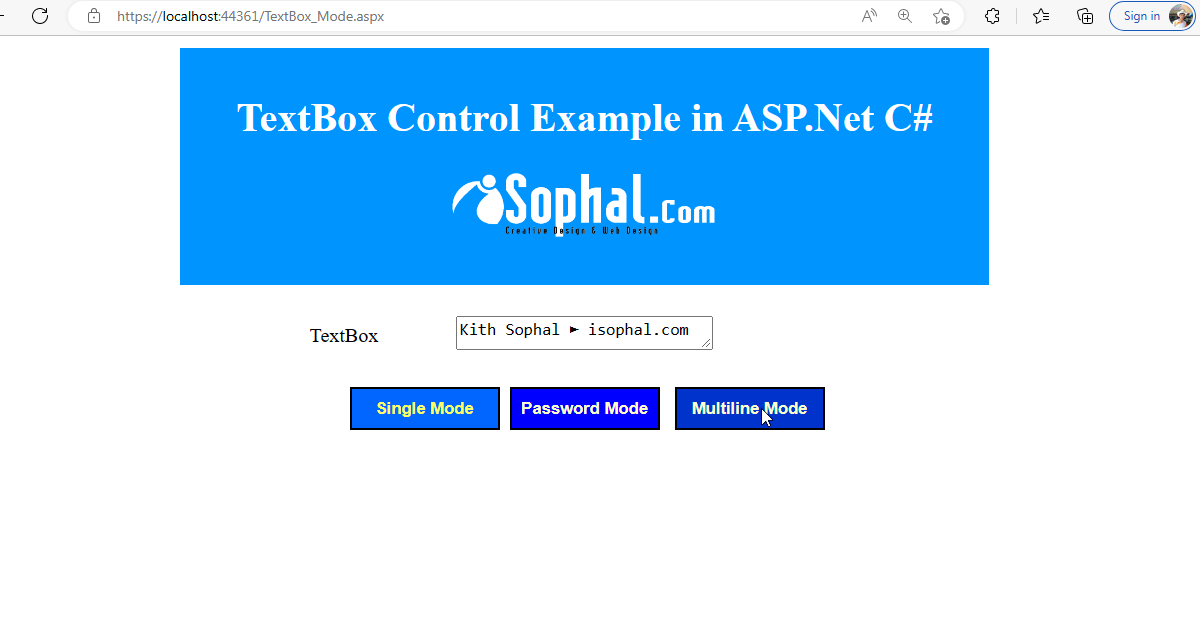
TextBox Control – ASP.Net Server Control
Textbox control is most usable web server control in asp.net.
TextBox control is a rectangular box which is used to take user to input. In simple word the TextBox is a place where user can input some text on asp.net web form. To use TextBox on page we can write code or just drag and drop from toolbox.
Syntax of ASP.Net TextBox
<asp:TextBox ID=”TextBox1″ runat=”server”></asp:TextBox>
All server side control has its own properties and events. below list shows textbox properties.
Properties | Description |
---|---|
ID | Identification name of textbox control. |
Text | It is used to display text in a control. |
BackColor | It is used to set background color of textbox control. |
ForColor | It is used to set text color of the control. |
ToolTip | It displays a text on control when mouse over on it. |
TabIndex | It is used manage tab order of control. |
CssClass | It is used to apply style on control. |
Enable | true/false – used to enable or disable control. |
Enable Theming | true/false – It is used to enable or disable effect of theme on control. |
CausesValidation | true/false – It is used to enable or disable validation effect on control |
Visible | true/false – It is used to hide or visible control on web page. |
Important Properties of TextBox control | |
MaxLenght | It is used to set maximum number of characters that can be input in TextBox. |
TextMode | Single / Multiline / Password |
ReadOnly | true/false – used to enable or disable control readonly. |
TextBox Example in ASP.Net
Above list shows the properties of TextBox control. now, lets understand more about control using example in C#.net.
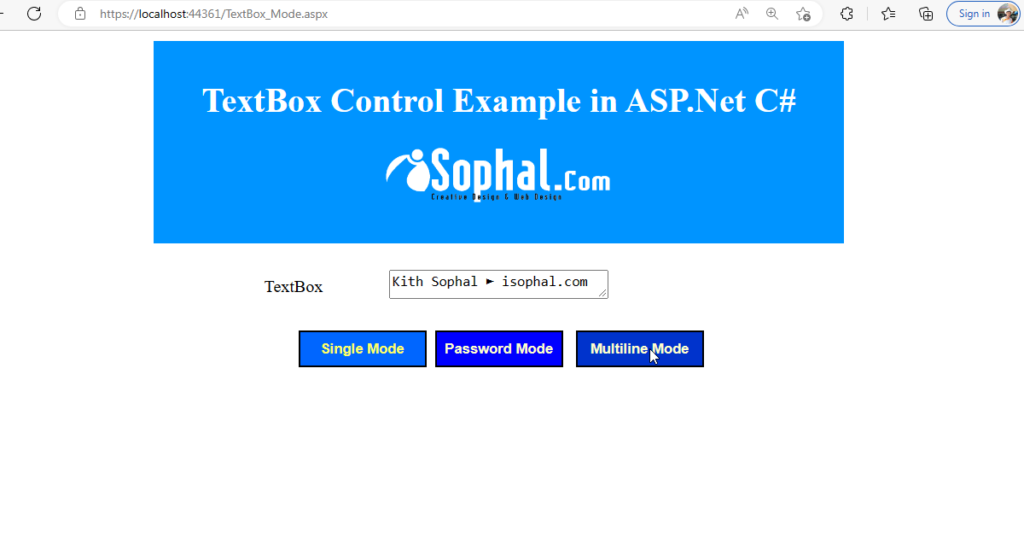
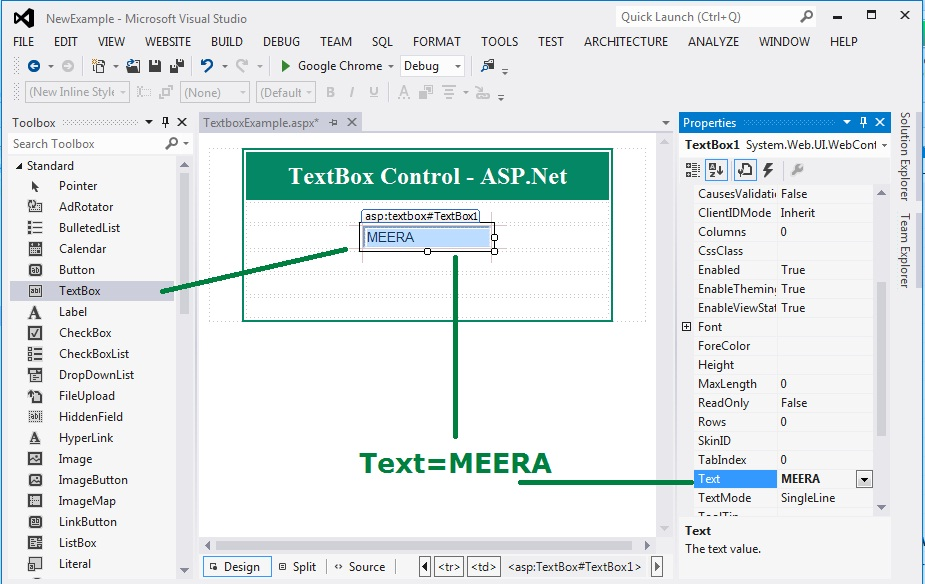
Assign value to and retrieve value from a TextBox control
TextBox1.Text = "ASP.Net with C#";
Label1.Text = TextBox1.Text;
TextBox Example
Assign a text to TextBox control when Button click event fires using c# in visual studio.
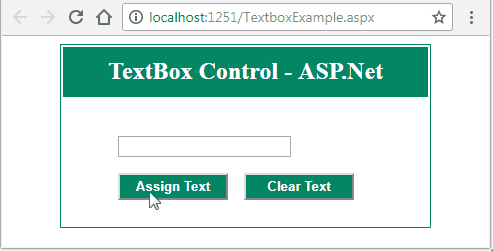
C# code for above TextBox example.
protected void btndisplay_Click(object sender, EventArgs e) {
TextBox1.Text = "MEERA";
}
protected void btnclear_Click(object sender, EventArgs e) {
TextBox1.Text = "";
}
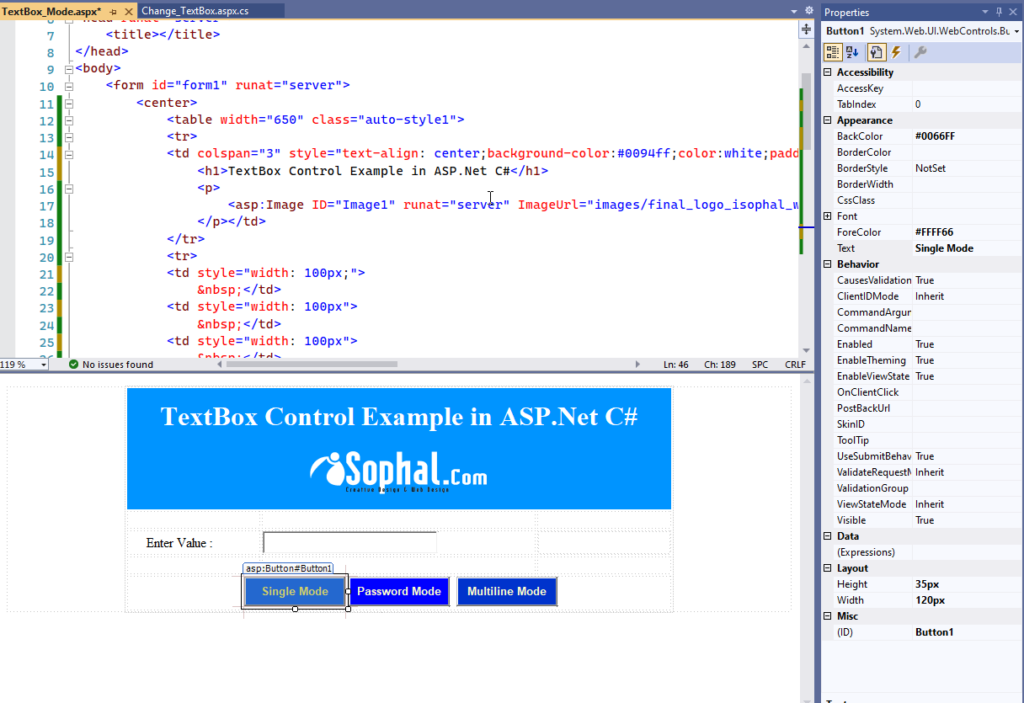
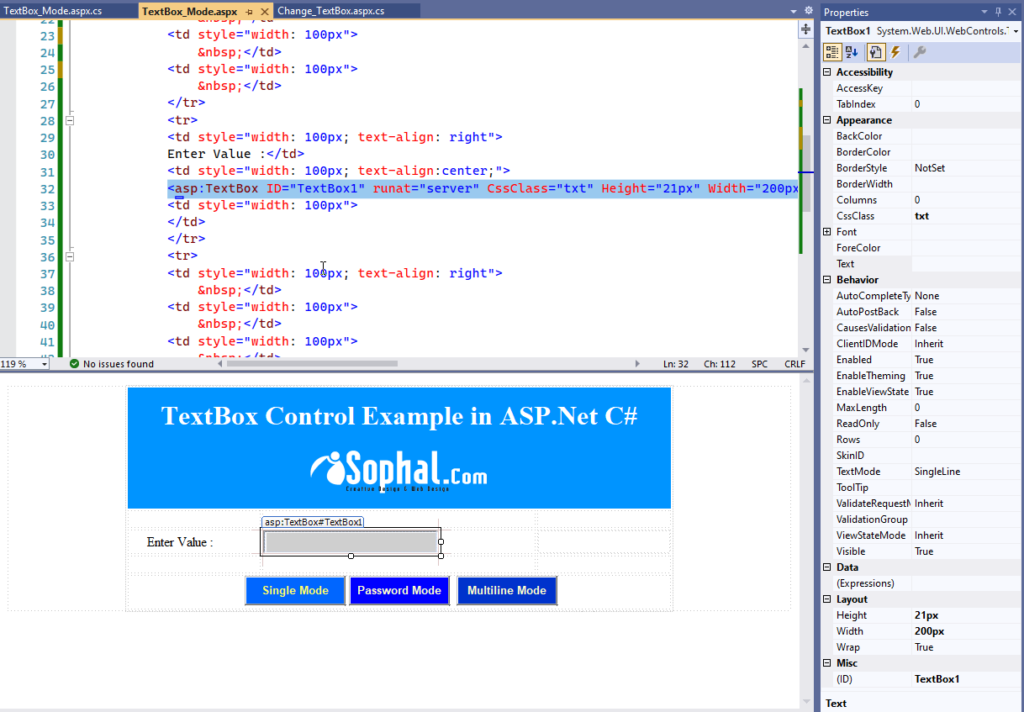
C# Code for above asp.net example
protected void Button1_Click(object sender, EventArgs e)
{
TextBox1.TextMode = TextBoxMode.SingleLine;
}
protected void Button2_Click(object sender, EventArgs e)
{
TextBox1.TextMode = TextBoxMode.Password;
}
protected void Button3_Click(object sender, EventArgs e)
{
TextBox1.TextMode = TextBoxMode.MultiLine;
}
TextBox Control Example
In this example we will change some textbox properties programmatically using c# in visual studio.
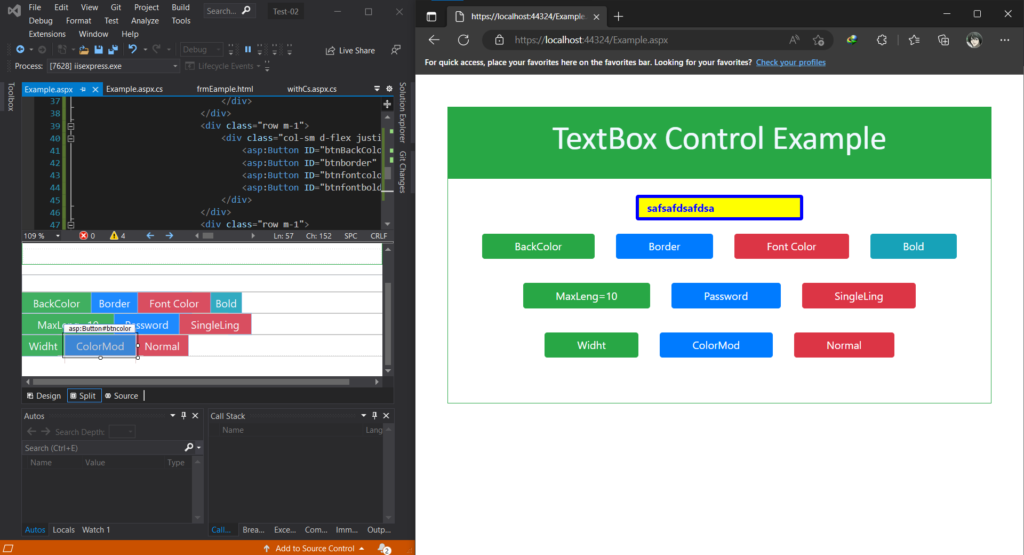
C# Code for above asp.net example
protected void btnbackcolor_Click(object sender, EventArgs e) {
TextBox1.BackColor = System.Drawing.Color.Yellow;
}
protected void btnborder_Click(object sender, EventArgs e) {
TextBox1.BorderStyle = BorderStyle.Solid;
TextBox1.BorderColor = System.Drawing.Color.Red;
TextBox1.BorderWidth = 3;
}
protected void btnfontcolor_Click(object sender, EventArgs e) {
TextBox1.ForeColor = System.Drawing.Color.Green;
}
protected void btnfontbold_Click(object sender, EventArgs e) {
TextBox1.Font.Bold = true;
}
protected void btn10_Click(object sender, EventArgs e) {
TextBox1.MaxLength = 10;
}
protected void btnpassword_Click(object sender, EventArgs e) {
TextBox1.TextMode = TextBoxMode.Password;
}
protected void btnsingleline_Click(object sender, EventArgs e) {
TextBox1.TextMode = TextBoxMode.SingleLine;
}
protected void btnwidth_Click(object sender, EventArgs e) {
TextBox1.Width = 200;
}
protected void btncolor_Click(object sender, EventArgs e) {
TextBox1.TextMode = TextBoxMode.Color;
}
protected void btnnormal_Click(object sender, EventArgs e) {
TextBox1.Text = "";
TextBox1.TextMode = TextBoxMode.SingleLine;
TextBox1.MaxLength = 0;
TextBox1.Font.Bold = false;
TextBox1.BackColor = System.Drawing.Color.White;
TextBox1.ForeColor = System.Drawing.Color.Black;
TextBox1.BorderStyle = BorderStyle.Solid;
TextBox1.BorderColor = System.Drawing.Color.Green;
TextBox1.BorderWidth = 1;
TextBox1.Width = 128;
}
We hope that this asp.net tutorial helped you to understand about TextBox control.