The DropDownList control is asp.net web server control. we can use dropdownlist control for hold group of items. The dropdownlist control is used to store the multiple items and allow user to select only one item from it.
The dropdownlist control is also known as combo box control. In dropdownlist control we can store multiple items but we can select only one item at a time, that’s why it is also known as Single Row Selection Box.
DropdownList Control Syntax :
<asp:DropDownListID=“DropDownList1“ runat=“server“></asp:DropDownList>
There is only one difference between dropdownlist control and listbox control – SelectionMode. The dropdownlist has no SelectionMode, so we can not select multiple item from dropdownlist.
DropDownList Control Example in ASP.Net C#
Here, we understand the dropdownlist control with an asp.net example.
Create a new web application in asp.net with a web forms. below screen shows how to take dropdownlist control on web page from toolbox.
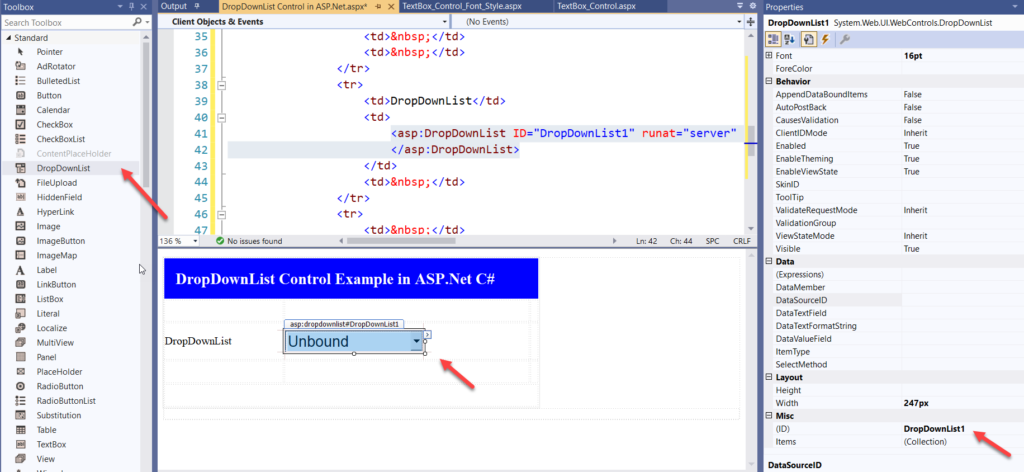
Now, for add new items in dropdownlist go to the Items property shows like below asp.net figure.
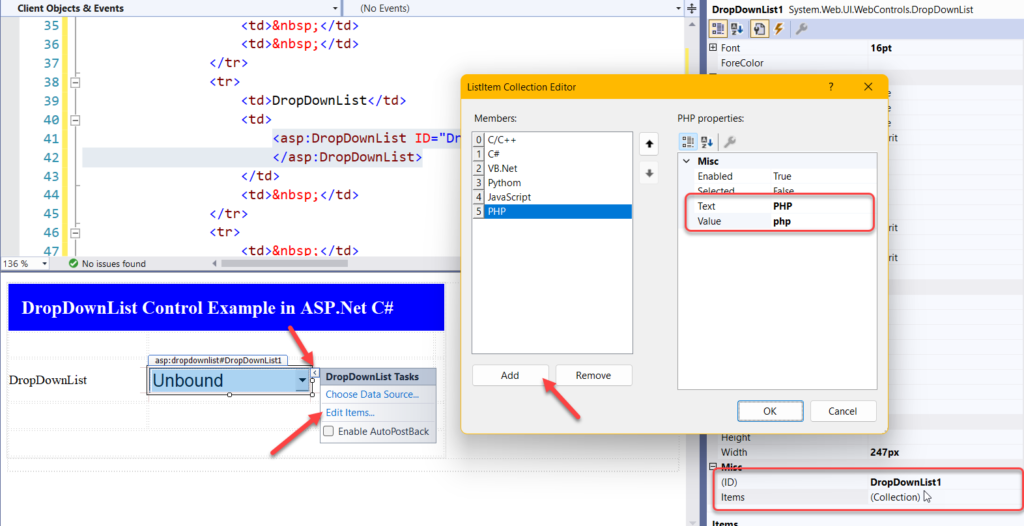
In dropdownlist control all the elements are known as items of dropdownlist, and each items consist with text and value attributes.
If we add items in to dropdwonlist control we must provide Text and Value for each items.
- Text = Text Property specify the Text display in the dropdownlist.
- Value = The value property is invisible value, but we can get the value while programming.
Here is the html code of dropdownlist control with five items.
<asp:DropDownList ID="DropDownList1" runat="server" Font-Size="16pt" Width="247px">
<asp:ListItem Value="1">C/C++</asp:ListItem>
<asp:ListItem Value="2">C#</asp:ListItem>
<asp:ListItem Value="3">VB.Net</asp:ListItem>
<asp:ListItem Value="4">Pythom</asp:ListItem>
<asp:ListItem Value="5">JavaScript</asp:ListItem>
<asp:ListItem Value="6">PHP</asp:ListItem>
</asp:DropDownList>
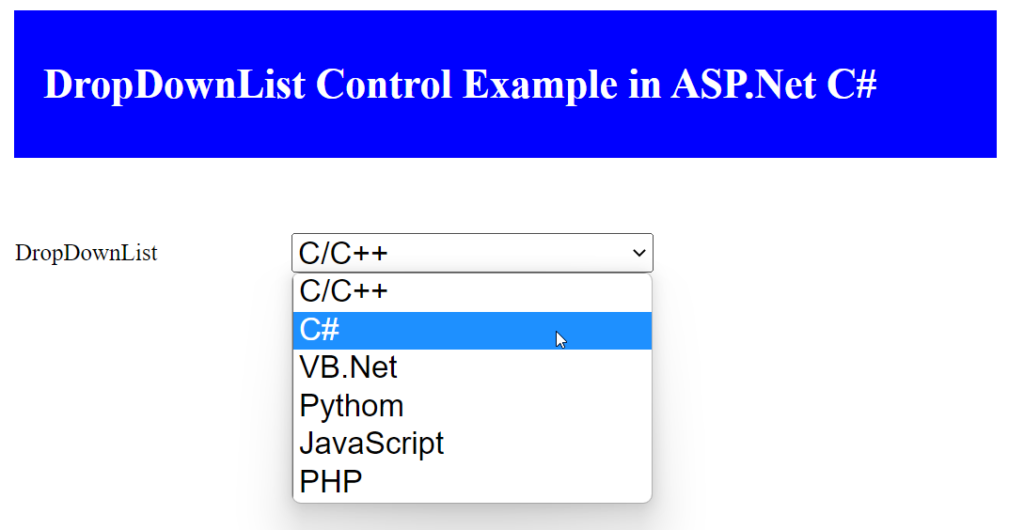
All server side control has its own properties and events. below list shows basic properties of Dropdownlist control.
Properties | Description |
---|---|
ID | Identification name of control. |
BackColor | It is used to set background color of control. |
ForColor | It is used to set text color of the control. |
ToolTip | It displays a text on control when mouse over on it. |
TabIndex | It is used manage tab order of control. |
CssClass | It is used to apply style on control. |
Enable | true/false – used to enable or disable control. |
Enable Theming | true/false – It is used to enable or disable effect of theme on control. |
CausesValidation | true/false – It is used to enable or disable validation effect on control |
Visible | true/false – It is used to hide or visible control on web page. |
Below lists show important properties of Dropdownlist control, which is use in programming side.
Properties | Description |
---|---|
Items | It shows the collection of item in the Dropdownlist. |
DropDownList1.Items.Count | Return the total count of items in the dropdownlist. |
DropDownList1.Items.Add(“ItemName”) | Add the new item in to the dropdownlist control. |
DropDownList1.Items.Insert(int index, “ItemName”) | Add the new item at specific position in dropdownlist control. |
DropDownList1.Items.Remove(“ItemName”) | Remove the item from the dropdownlist control. |
DropDownList1.Items.RemoveAt(int index) | Remove the item from the dropdownlist at desired position index. |
DropDownList1.Items.Clear() | Clear all the items from dropdownlist control. |
DropDownList1.SelectedItem.Text | Returns the Text value of selected item of the dropdownlist. |
DropDownList1.SelectedValue | Returns the Value property of the selected item of the dropdownlist. |
DropDownList1.SelectedIndex | Returns the Index of selected item of dropdownlist. (Index always start from Zero). |
Now, let’s see all programming properties with C# Example.
DropDownList1.Items.Count
Below example show the total number of item count in dropdownlist control.
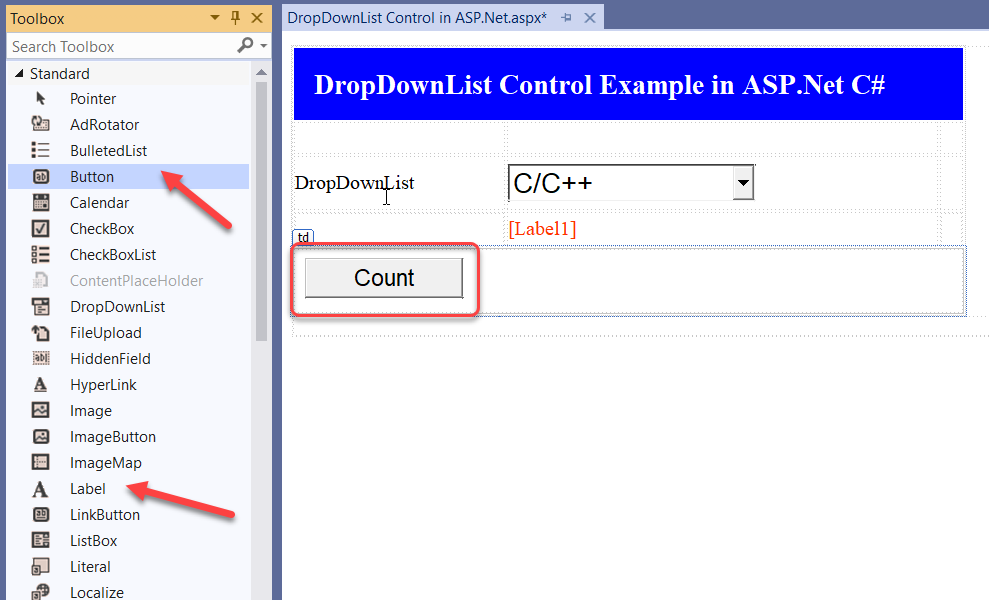
protected void btncount_Click(object sender, EventArgs e)
{
Label1.Text = "The Count = " + DropDownList1.Items.Count.ToString();
}
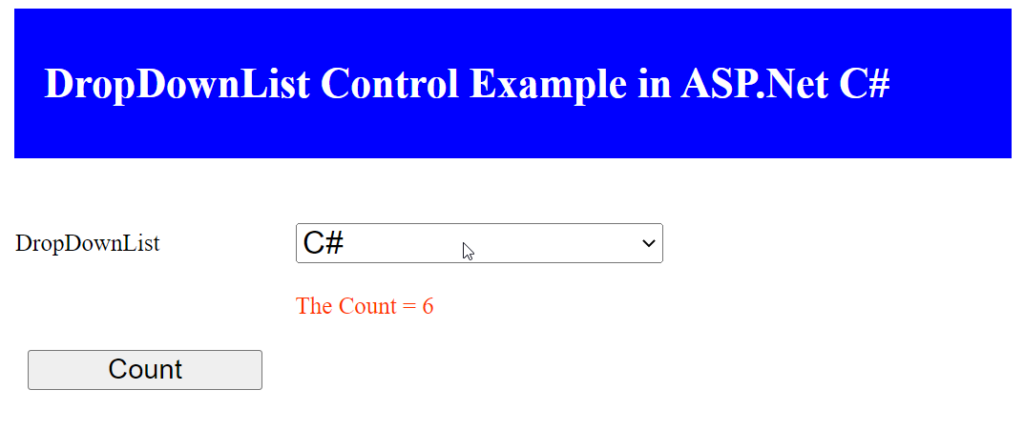
DropDownlList1.SelectedItem.Text
Example : How to get selected item from dropdownlist control in asp.net.
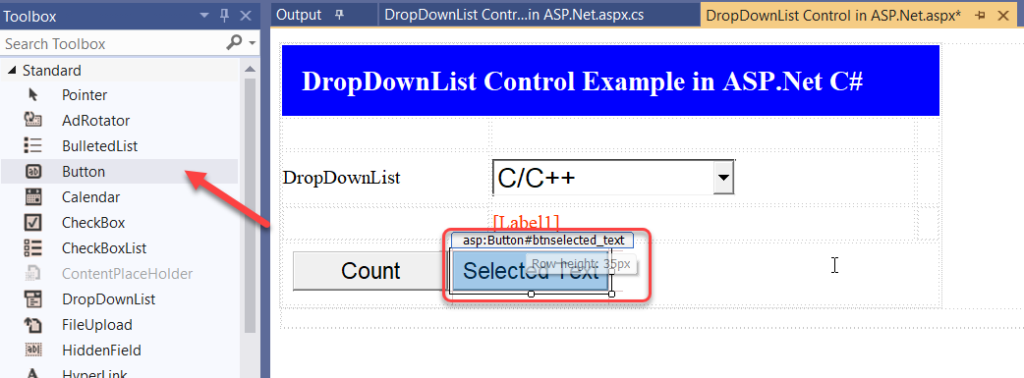
protected void btnselected_text_Click(object sender, EventArgs e)
{
Label1.Text = "Text = " + DropDownList1.SelectedItem.Text;
}
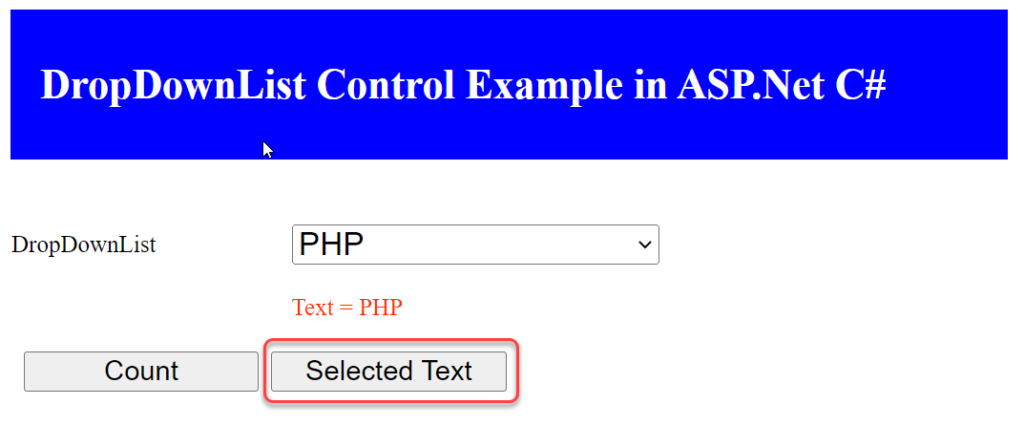
DropDownList1.SelectedValue
Example : How to get Selected Value from dropdownlist control in asp.net.
protected void btnselected_value_Click(object sender, EventArgs e)
{
Label1.Text = "Value = " + DropDownList1.SelectedValue;
}
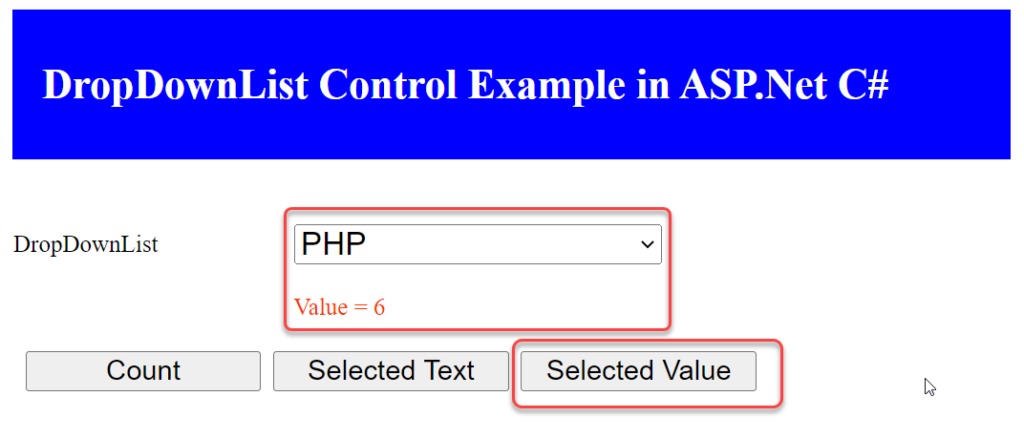
DropDownList1.SelectedIndex
Example: Get Selected Index in dropdownlist control in asp.net.
protected void btn_index_Click(object sender, EventArgs e)
{
Label1.Text = "Index = " + DropDownList1.SelectedIndex.ToString();
}
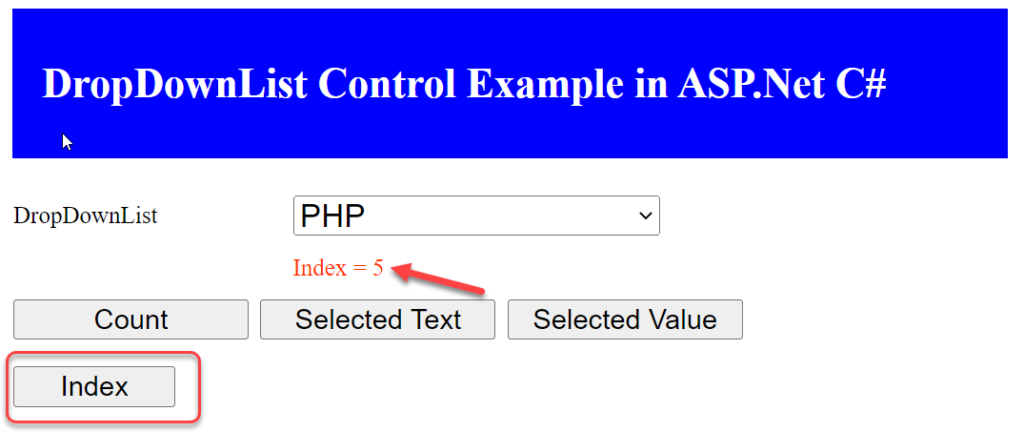
DropDownList1.Items.Clear()
Example : Clear all items in dropdownlist control.
protected void btn_clear_Click(object sender, EventArgs e)
{
DropDownList1.Items.Clear();
Label1.Text = "ListBox Cleared";
}
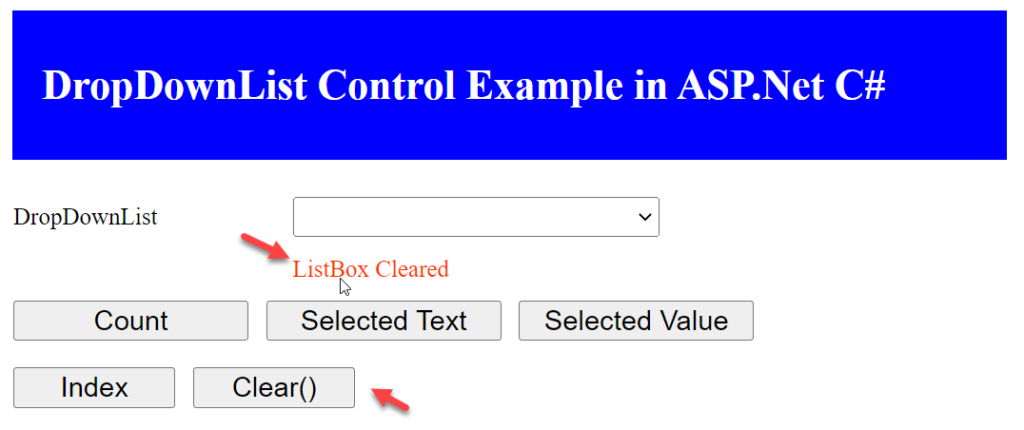
DropDownList1.Items.Add(“text”);
Example : Add new item in dropdownlist control.
protected void btn_add_Click(object sender, EventArgs e)
{
DropDownList1.Items.Add("ASP.Net");
Label1.Text = "Item Added";
}
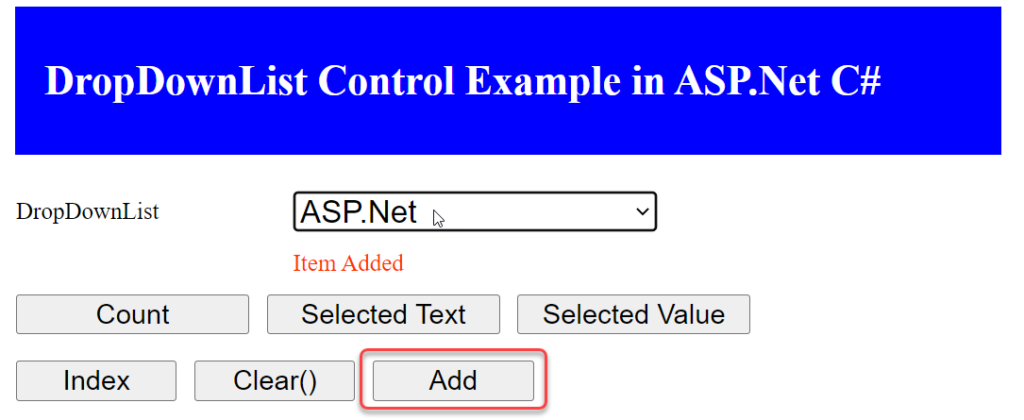
DropDownList1.Items.Remove(“text”)
Example : Remove items from dropdownlist control.
protected void btn_remove_Click(object sender, EventArgs e)
{
DropDownList1.Items.Remove("PHP");
Label1.Text = "Item Removed";
}
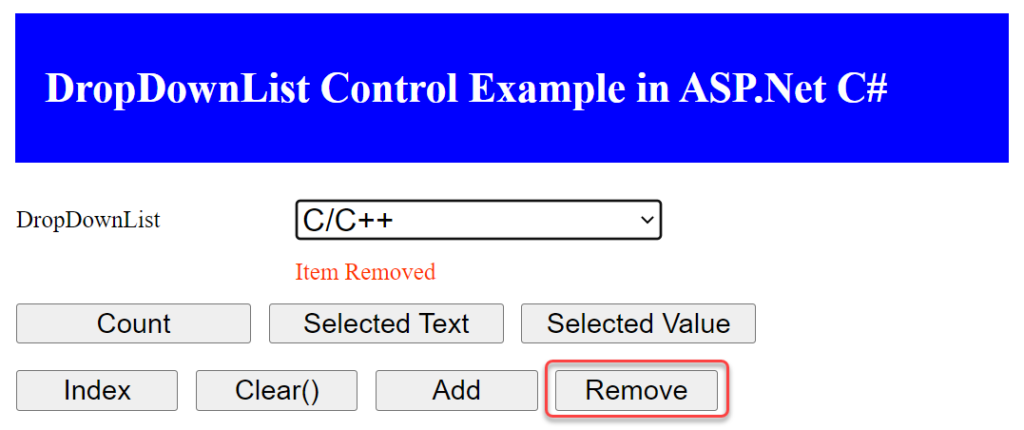
DropDownList1.Items.Insert(int index, “text”);
Example : Add new item at specific location in dropdownlist control. The index is the indicate the location of newly added item in dropdownlist control.
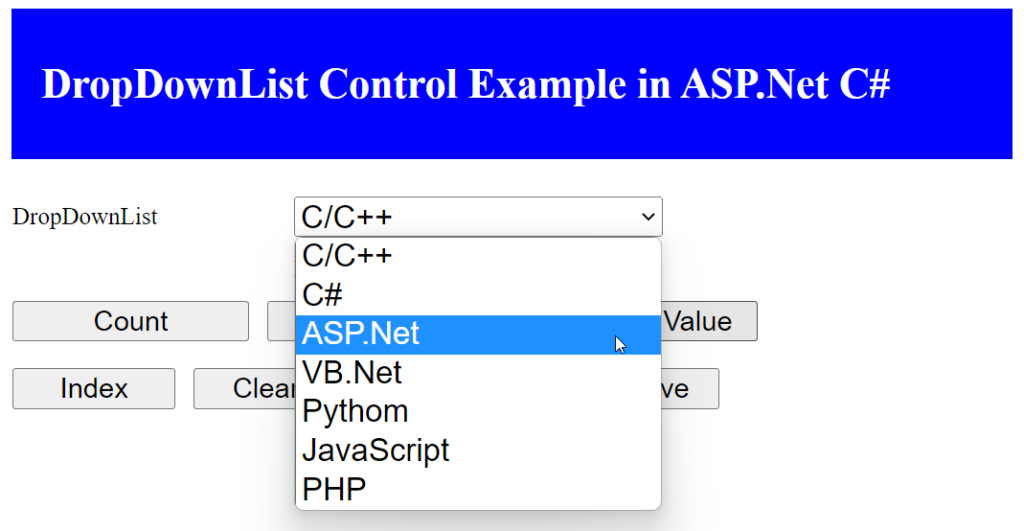
protected void btn_add_Click(object sender, EventArgs e)
{
DropDownList1.Items.Insert(2, "ASP.Net");
Label1.Text = "Item Inserted";
}
DropDownList1.Items.RemoveAt(int index)
Example : Remove items from dropdownlist control at specify the index location. The index start from zero.
protected void btn_remove_Click(object sender, EventArgs e)
{
DropDownList1.Items.RemoveAt(2);
Label1.Text = "Item Removed";
}
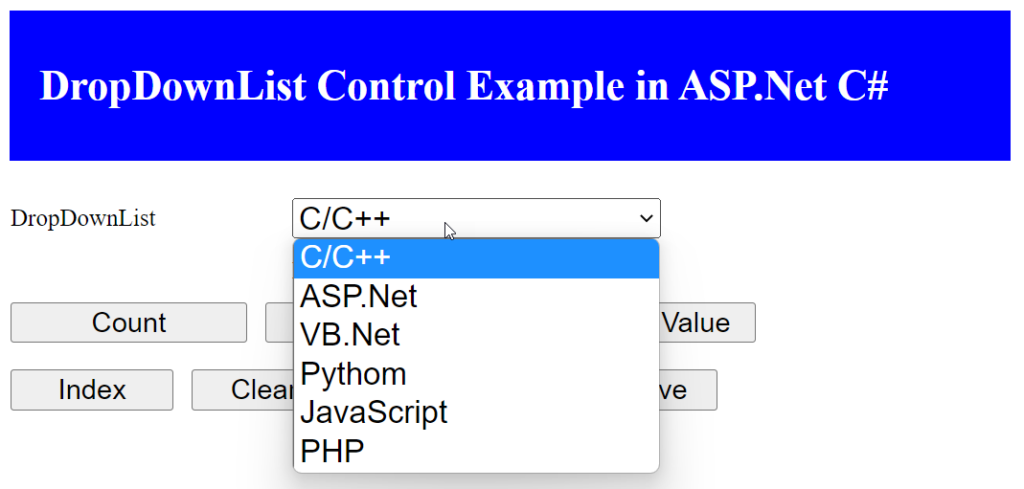
The C# code behind code for all buttons click events.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace D2ASPdoNet
{
public partial class DropDownList_Control_in_ASP_Net : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btncount_Click(object sender, EventArgs e)
{
Label1.Text = "The Count = " + DropDownList1.Items.Count.ToString();
}
protected void btnselected_text_Click(object sender, EventArgs e)
{
Label1.Text = "Text = " + DropDownList1.SelectedItem.Text;
}
protected void btnselected_value_Click(object sender, EventArgs e)
{
Label1.Text = "Value = " + DropDownList1.SelectedValue;
}
protected void btn_index_Click(object sender, EventArgs e)
{
Label1.Text = "Index = " + DropDownList1.SelectedIndex.ToString();
}
protected void btn_clear_Click(object sender, EventArgs e)
{
DropDownList1.Items.Clear();
Label1.Text = "ListBox Cleared";
}
protected void btn_add_Click(object sender, EventArgs e)
{
DropDownList1.Items.Insert(2, "ASP.Net");
Label1.Text = "Item Inserted";
}
protected void btn_remove_Click(object sender, EventArgs e)
{
DropDownList1.Items.RemoveAt(2);
Label1.Text = "Item Removed";
}
}
}
We hope that this asp.net tutorial helped you to understand about DropDownList control.