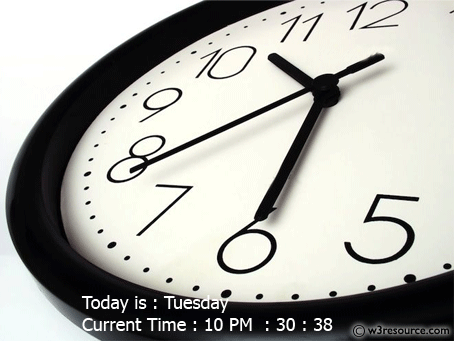
Print the content of a div element using JavaScript
Example 1: This example uses JavaScript window print command to print the content of div element.
Sample Solution:
Print the content of a div
<!-- Script to print the content of a div -->
<script>
function printDiv() {
var divContents = document.getElementById("GFG").innerHTML;
var a = window.open('', '', 'height=500, width=500');
a.document.write('<html>');
a.document.write('<body > <h1>Div contents are <br>');
a.document.write(divContents);
a.document.write('</body></html>');
a.document.close();
a.print();
}
</script>
<div id="GFG" style="background-color: green;">
<h2>Geeksforgeeks</h2>
<p>
This is inside the div and will be printed
on the screen after the click.
</p>
</div>
<input type="button" value="click" onclick="printDiv()">
Output
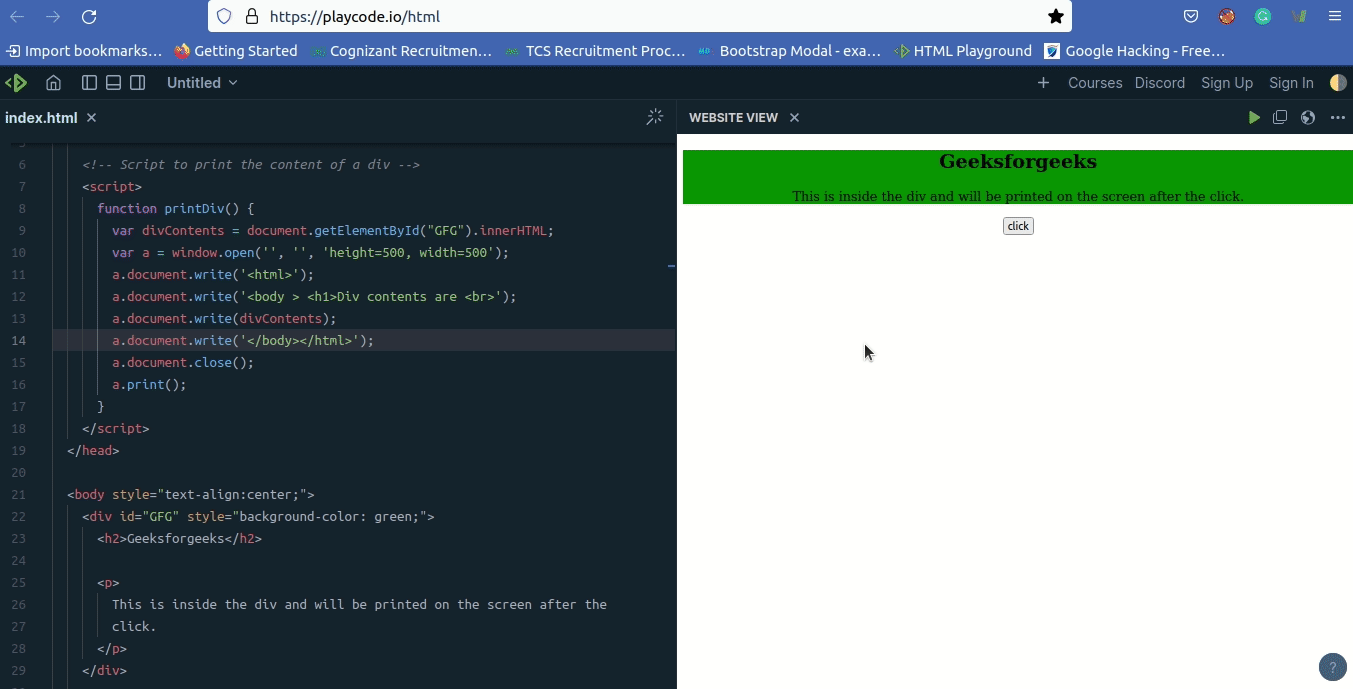
How to create a pop-up to print dialog box using JavaScript?
<!DOCTYPE html>
<html>
<head>
<title>create a pop-up to print
dialog box using JavaScript</title>
</head>
<body>
<center>
<h1 style="color:rgb(255, 174, 0)">Print dialog box using JavaScript</h1>
<script>
function printPopUp() {
alert("Pop-up dialog-box")
window.print();
}
</script>
<button onclick="printPopUp()">Print</button>
</center>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>create a pop-up to print
dialog box using JavaScript</title>
</head>
<body>
<center>
<h1 style="color:rgba(255, 136, 0, 0.918)">
Print dialog box using JavaScript
</h1>
<a href="javascript:alert('Pop-up dialog-box');window.print();">
Click Me
</a>
</center>
</body>
</html>