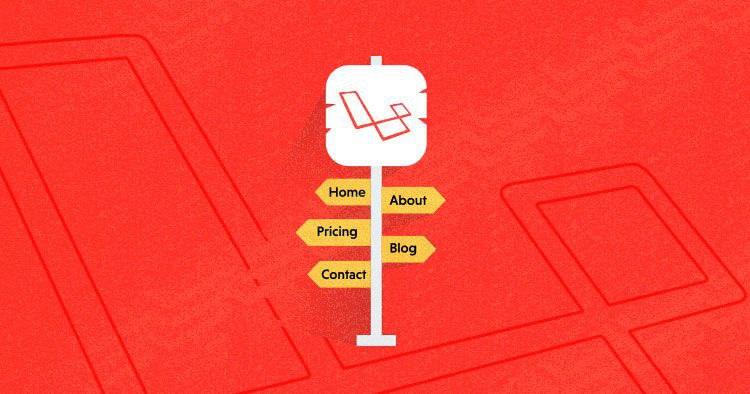
An Introduction to Routing in Laravel 10.x
Route
What is a Route?
ការប្រើប្រាស់ Route ក្នុង Laravel framework ។ Route គឺវាមានលក្ខណះសំខាន់ណាស់សំរាប់ Laravel framework ពីព្រោះវាជាអ្នកកំណត់នៅពេលដែលយើងធ្វើ request root directory website ថាតើត្រូវបង្ហាញ Page មួយណាចឹង ហើយ Route គឺជាអ្នកកំនត់ហើយទីតាំងរបស់ Rout គឺស្ថិតនៅក្នុង៖
In web frameworks, a route is a way of defining a URL pattern that the framework should respond to. In Laravel, routes are used to map a URL to a specific action (e.g., loading a view, running a controller method).
Basic Route
How to Create Routes in Laravel
- Open routes/web.php and define a basic route:
use Illuminate\Support\Facades\Route;
Route::get(‘/’, function () {
return ‘Hello, World!’;
});

Multiple Route នឹង Parameters Route
- Rout Parameter គឺវាក៏មានភាពចាំបាច់ក្នុងការបញ្ចូន Data ដូចជា Id សំរាប់យក Id ទៅធ្វើលក្ខខណ្ឌដូចជា លុប កែប្រែ ជាដើម។
ឧទារណ៍៖ Multiple Route
//route multiple
Route::match([‘get’,’post’],’/match‘, function () {
return (‘This is match for Multiple Route & Parameters Route’);
});


Multiple Route នឹង Parameters Route
Route Multiple
ការប្រើប្រាស់ Validate Route Parameters
- Route Multiple
គឺមានន័យថាពេលដែលលោកអ្នកបញ្ចូល Method get នឹង Post វានឹងធ្វើពេលដែលលោកអ្នក request មក match មួយនឹង។
ឧទារណ៍៖ route parameter
//route parameter
Route::get(‘/view/{id}’, function ($id) {
return ‘This is view id=’.$id;
});
Output:
http://127.0.0.1:8000/view/2023
Display:
This is view id=2023
ឧទារណ៍ ១៖
I will now create a basic route that will print the table of 2.
Route::get(‘/table’, function () {
for($i =1; $i <= 10 ; $i++){
echo “$i * 2 = “. $i*2 .”<br>”;
}
});
Output:
http://127.0.0.1:8000/table
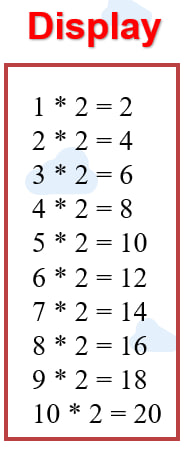
ឧទារណ៍ ២៖
Now, what if I want the user to decide the number for which the route will print the table? Here is the code for such a route:
Route::get(‘/table/{number}’, function ($number) {
for($i =1; $i <= 10 ; $i++){
echo “$i * $number = “. $i* $number .”<br>”;
}
});
Output:
http://127.0.0.1:8000/table/9
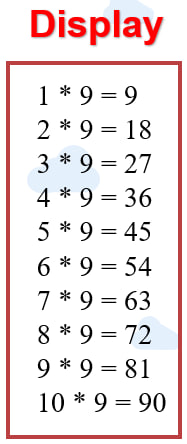
ឧទារណ៍ ៣៖
Regular Expressions Constraints For Route Parameters
Route::get(‘/table/{number?}’, function ($number = 2) {
for($i =1; $i <= 10 ; $i++){
echo “$i * $number = “. $i* $number .”<br>”;
}
})->where(‘number’, ‘[0-9]+’);
Output:
http://127.0.0.1:8000/table/9
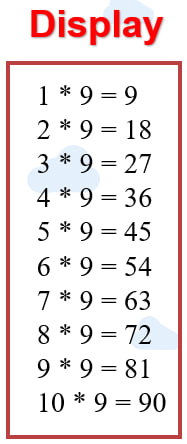
ឧទារណ៍ ៤៖
Multiple parameter pass through Route & URL in Laravel
Route::get(‘sum/{n1}/{n2}/{n3}’, function (int $n1, int $n2, int $n3) {
$total = $n1 + $n2 + $n3;
return “Total =”.$total;
})->name(‘sum’);
Output:
http://127.0.0.1:8000/sum/21/22/13
Display:Total =56
ឧទារណ៍ ៥៖
To create multiple parameters dynamic routes in Laravel 10.x, you can use the Route::get() method with curly braces to enclose the parameters. For example, the following code will create a route with two parameters:
Route::get(‘user/{userid}/post/{postid}’,function (int $userid, int $postid) {
return “User ID: {$userid}, Post ID: {$postid}”;
});
Output:
http://127.0.0.1:8000/user/20/post/25
Display:
User ID: 20, Post ID: 25
ការប្រើប្រាស់ Routing ► View | Laravel 10.x
ឧទារណ៍ ១៖
Regular Expressions Constraints for Route Parameters
//validate rout Parameters
Route::get(‘user/{id}’, function ($id) {
return ‘User ID: ‘ . $id;
})->where(‘id’, ‘[0-9]+’);
http://127.0.0.1:8000/user/23 (Correct)
http://127.0.0.1:8000/user/abc (Incorrect)
In the above route, {id} must be a number. If you try accessing /user/abc, it won’t match this route.
ឧទារណ៍ ២៖ Validate Route Parameters
លោកអ្នកអាចធ្វើការ កាពារមិនអោយគេបញ្ចូល Parameter ជាអក្សបានដោយប្រើប្រភេទ Route ដូចខាងក្រោម។
//validate rout Parameters
Route::get(‘/view/{id}’, function ($id) {
return ‘This is view id=’.$id;
})->where(‘id’,'[0-9]+’);
http://127.0.0.1:8000/view/2023 (Correct)
http://127.0.0.1:8000/view/bbu (Incorrect)
ចំនាំ៖ Where id មានន័យថាលោកអ្នកត្រូវធ្វើការបញ្ចូល Id បានជាលេខប៉ុន្នោះ ប្រសិនបើបញ្ចូលខុសពីលេកវានឹង Error។
ឧទារណ៍ ៣៖ Validate Route Parameters | Email
//validate rout Parameters | Email
Route::get(’email/{email}’, function (string $email) {
return “Email: {$email}”;
})->where(’email’, ‘[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]+’);
http://127.0.0.1:8000/email/sophal@gmail.com (Correct)
http://127.0.0.1:8000/email/sophal (Incorrect)
Display:
Email: sophal@gmail.com
ការប្រើប្រាស់ Routing ► View | Laravel 10.x
- ស្វែងយល់ពី Layout (Blade Templates) នៅក្នុង Laravel Framework
- Blade ត្រូវបានធ្វើការ Compile ជាមួយ PHP Code ធម្មតារហូតទាល់តែវាត្រូវបានដំណើរការចប់ ។ Blade view file .blade.php ត្រូវបានគេហៅថាជា extension ឬ ជាប្រភេទ File ដែលបាន Store ទុកនៅក្នុង resources/views File។
Creating a View:
- Create a new Blade view file: resources/views/welcome.blade.php. Add the following content:
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Welcome to Laravel Views</title>
</head>
<body>
<h1>Hello from Laravel Views!</h1>
</body> </html>
Route to a View:
- Open routes/web.phpand add:
Route::get(‘/homepage’, function () {
return view(‘home’);
});
Output:
http://127.0.0.1:8000/homepage
Display:
Hello from Laravel Views!