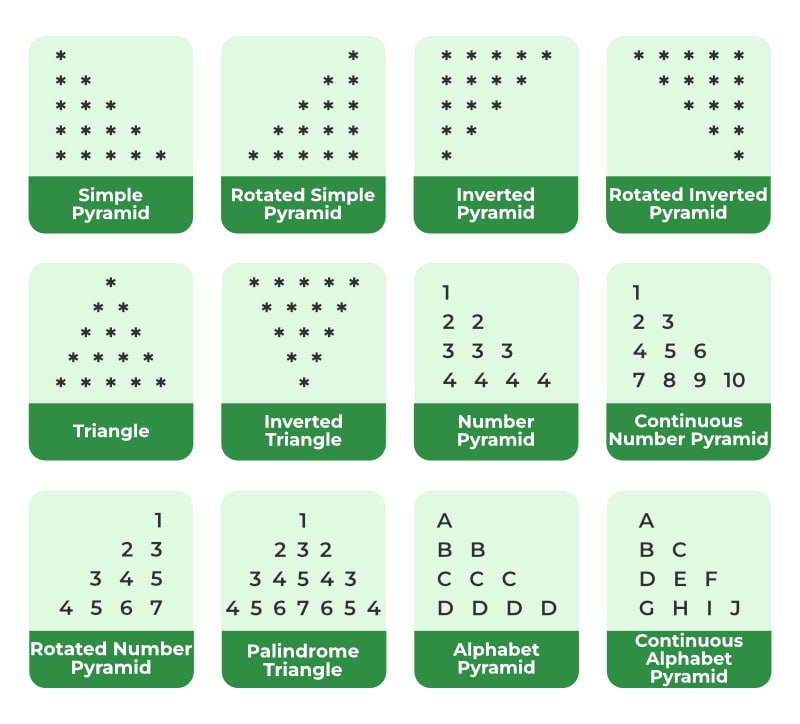
Star Patterns Program in C
We are going to cover the following patterns. You can view the code of any pattern given below by clicking on the pattern.
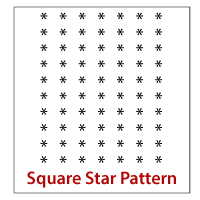
Square Star Pattern
The code to create the square star pattern is given below:
- #include <stdio.h>
- int main()
- {
- int n;
- printf(“Enter the number of rows”);
- scanf(“%d”,&n);
- for(int i=0;i<n;i++)
- {
- for(int j=0;j<n;j++)
- {
- printf(“*”);
- }
- printf(“\n”);
- }
- return 0;
- }
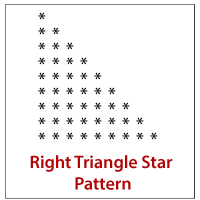
Hollow Mirrored Rhombus Star Pattern
The code for the hollow mirrored rhombus star pattern is given below:
- #include <stdio.h>
- int main()
- {
- int n;
- printf(“Enter the number of rows”);
- scanf(“%d”,&n);
- for(int i=1;i<=n;i++)
- {
- for(int j=1;j<i;j++)
- {
- printf(” “);
- }
- for(int k=1;k<=n;k++)
- {
- if(i==1 || i==n || k==1 || k==n)
- printf(“*”);
- else
- printf(” “);
- }
- printf(“\n”);
- }
- return 0;
- }
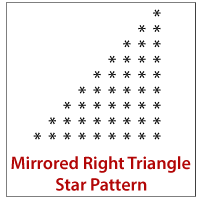
Right Triangle Star Pattern
- #include <stdio.h>
- int main()
- {
- int n;
- printf(“Enter the number of rows”);
- scanf(“%d”,&n);
- for(int i=1;i<=n;i++)
- {
- for(int j=1;j<=i;j++)
- {
- printf(“* “);
- }
- printf(“\n”);
- }
- return 0;
- }
Output
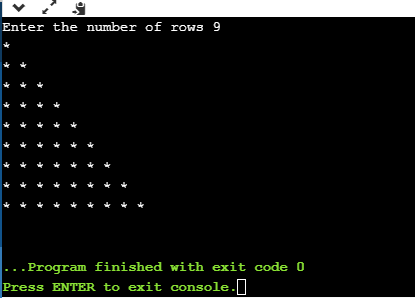
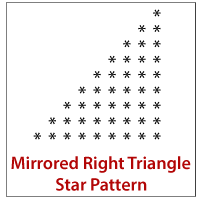
Mirrored Right Triangle Star Pattern
The code for the mirrored right triangle star pattern is given below:
- #include <stdio.h>
- int main()
- {
- int n,m=1;
- printf(“Enter the number of rows”);
- scanf(“%d”,&n);
- for(int i=n;i>=1;i–)
- {
- for(int j=1;j<=i-1;j++)
- {
- printf(” “);
- }
- for(int k=1;k<=m;k++)
- {
- printf(“*”);
- }
- printf(“\n”);
- m++;
- }
- return 0;
- }
Output
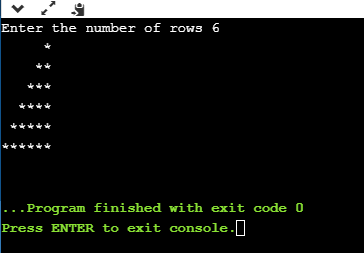
Pyramid Star Pattern
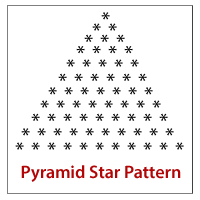
- #include <stdio.h>
- int main()
- {
- int n,m;
- printf(“Enter the number of rows”);
- scanf(“%d”,&n);
- m=n;
- for(int i=1;i<=n;i++)
- {
- for(int j=1;j<=m-1;j++)
- {
- printf(” “);
- }
- for(int k=1;k<=2*i-1;k++)
- {
- printf(“*”);
- }
- m–;
- printf(“\n”);
- }
- return 0;
- }
Output
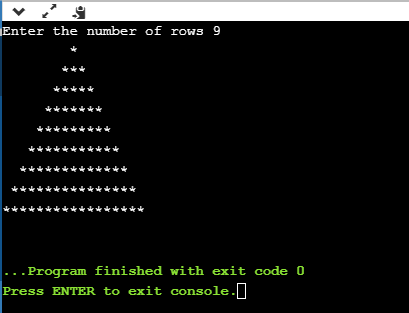
Inverted Pyramid Star Pattern
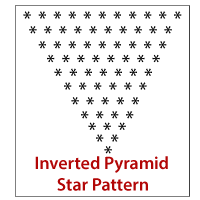
- #include <stdio.h>
- int main()
- {
- int n,m=1;
- printf(“Enter the number of rows”);
- scanf(“%d”,&n);
- for(int i=n;i>=1;i–)
- {
- for(int j=1;j<m;j++)
- {
- printf(” “);
- }
- for(int k=1;k<=2*i-1;k++)
- {
- printf(“*”);
- }
- m++;
- printf(“\n”);
- }
- return 0;
- }
Output
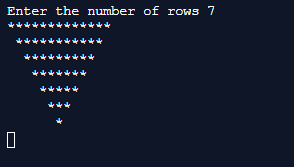
Right Triangle Star Pattern
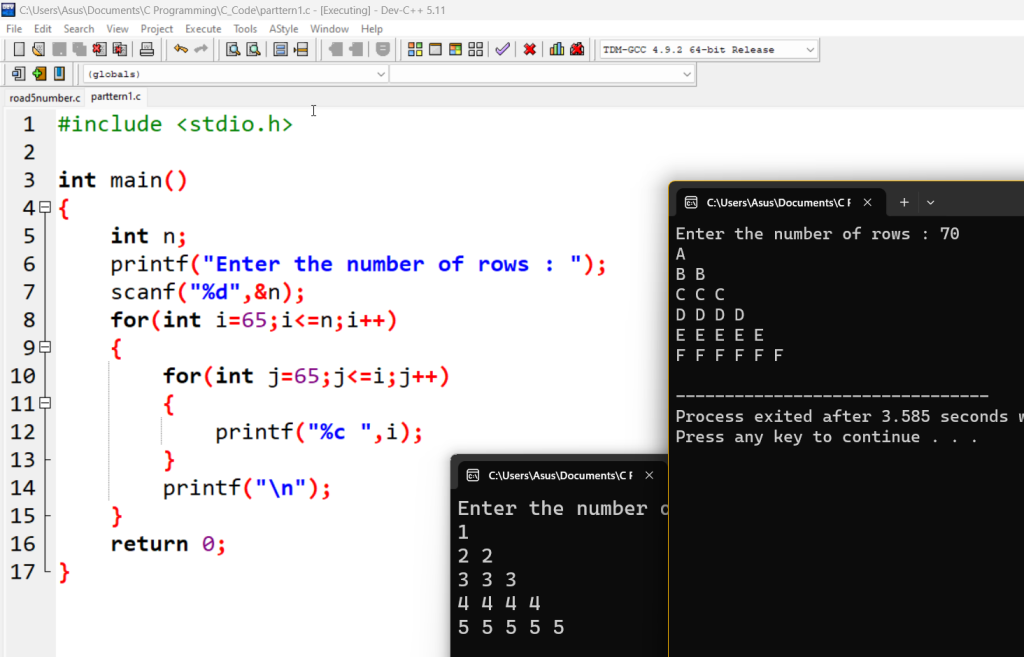
include
int main()
{
int n;
printf(“Enter the number of rows : “);
scanf(“%d”,&n);
for(int i=65;i<=n;i++)
{
for(int j=65;j<=i;j++)
{
printf(“%c “,i);
}
printf(“\n”);
}
return 0;
}