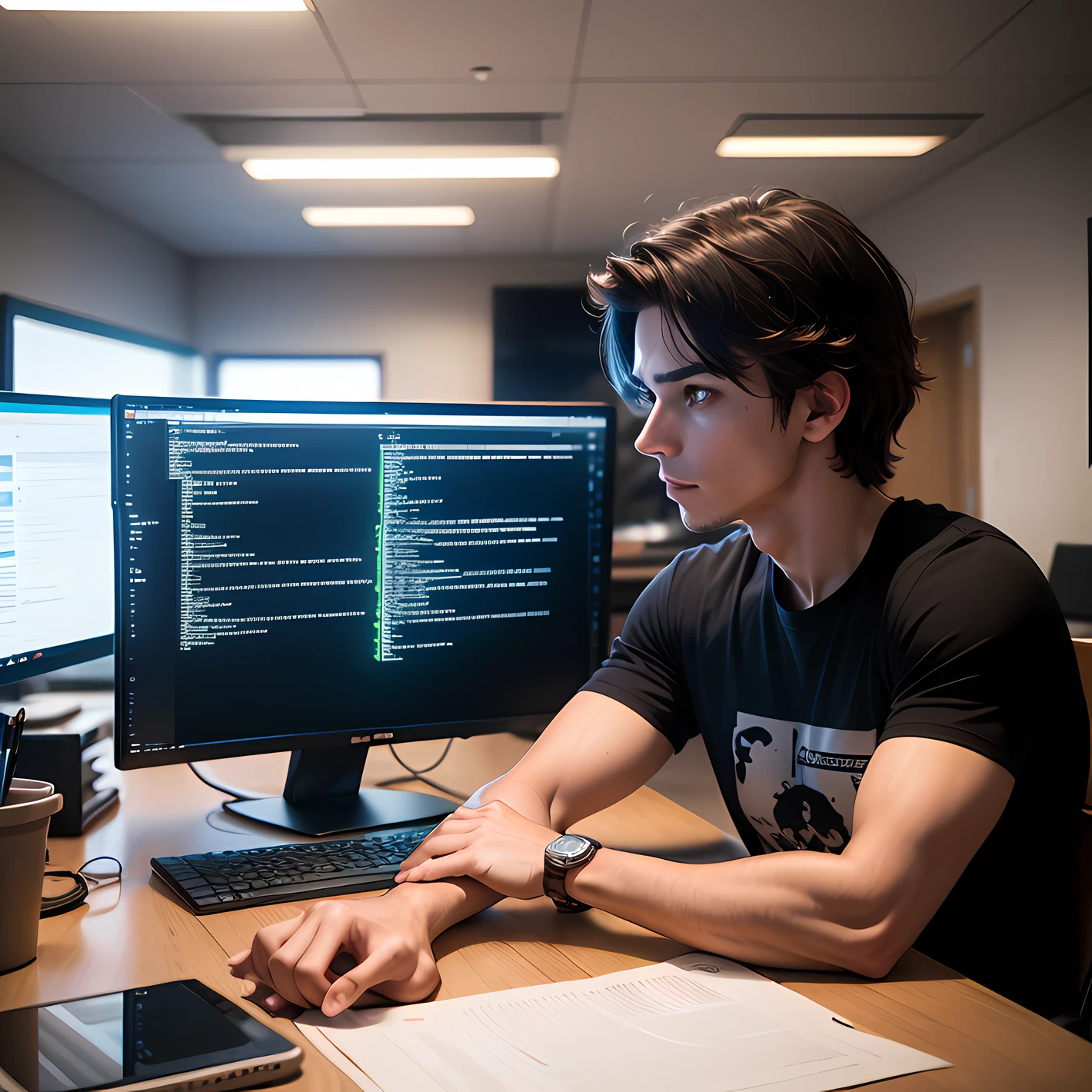
ការប្រើប្រាស់ Functions ក្នុងភាសា C
Functions គឺជាបណ្ដុំកូដ ដែលដំណើរការការងារជាក់លាក់មួយ។
យើងអាចបង្កើត function មួយដោយខ្លួនឯងបាន គឺអាស្រ័យដោយ function ចំនួនបី នោះគឺ៖
- Function Declaration : ជា function ដែលត្រូវបានប្រកាសជា global នៅពីលើ main function ។ យើងបង្កើត function នេះឡើងដើម្បីប្រាប់ compiler ឳ្យដឹងថា តើ function ដែលយើងនឹងបង្កើត គឺជា function ប្រភេទអ្វី (function with parameter, return type) ហើយមានឈ្មោះអ្វី?
- Function Call : គឺជា function មួយដែលយើងបង្កើតវាឡើងដើម្បីហៅ function ដែលយើងបានបង្កើតយកមកប្រើ ដើម្បីឳ្យវាដំណើរការនៅកន្លែងដែលយើងបានហៅនោះ ។
- Function Definition : ជា function ដែលផ្ទុកនូវ Code ដែលយើងបានសរសេរទុកសម្រាប់ដំណើរការ។
- Return Function: ជា function ដែលអាច return/ផ្ទេរ តម្លៃពី function definition ទៅឳ្យ function call។ នៅក្នុង return function យើងនឹងសិក្សាទៅលើលក្ខណៈពីរបន្ថែមទៀតគឺ Return Function with Parametersនិង Return Function with no parameters។
- Non-Return Function: ជា function ដែលមិនអាច return តម្លៃចេញទៅឳ្យ function ផ្សេងបានទេ។ នៅក្នុង non-return function ក៏មានលក្ខណៈពីរផងដែរគឺ Non-Return Function with Parameters និង Non-Return Function with no parameters។ ជាទូទៅ non-return function គឺផ្ដើមដោយ keyword “void”។
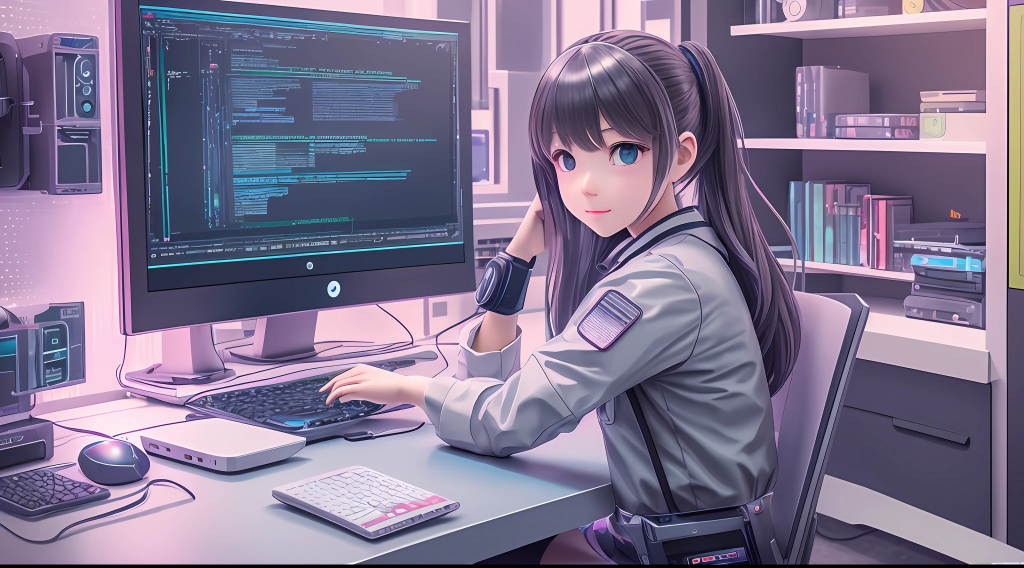
In C programming, a function is defined as a self-contained block of statements that perform a coherent task of some kind. Every C program can be designed in terms of functions, and the return
statement in a function is used to exit from the function and go back to the point from where it was called. The return
statement can also return a value from the function to the calling function.
Here is a simple example to illustrate how functions and the return
statement work in C:
Example: Function to Add Two Numbers
#include <stdio.h>
// Function declaration
int addNumbers(int a, int b);
int main() {
int num1, num2, sum;
// Input numbers from user
printf("Enter two numbers: ");
scanf("%d %d", &num1, &num2);
// Function call
sum = addNumbers(num1, num2);
// Output the returned value
printf("Sum = %d", sum);
return 0;
}
// Function definition
int addNumbers(int a, int b) {
int result;
result = a + b;
// Return statement
return result;
}
Explanation:
- Function Declaration: The function
addNumbers(int a, int b)
is declared before themain()
function. This declaration tells the compiler about the function’s name, return type, and parameters. - Function Call: Inside the
main()
function,addNumbers
is called withnum1
andnum2
as arguments. The function computes the sum of these numbers and returns it. - Return Statement: The
return result;
statement inaddNumbers
function sends the value ofresult
back to the caller, which is then assigned to the variablesum
in themain()
function. - Output: The program prompts the user to enter two numbers, computes their sum through a function call, and prints the result.
This example demonstrates how functions can be used to organize and reuse code effectively in C programming, as well as how values can be returned from a function to the calling code using the return
statement.
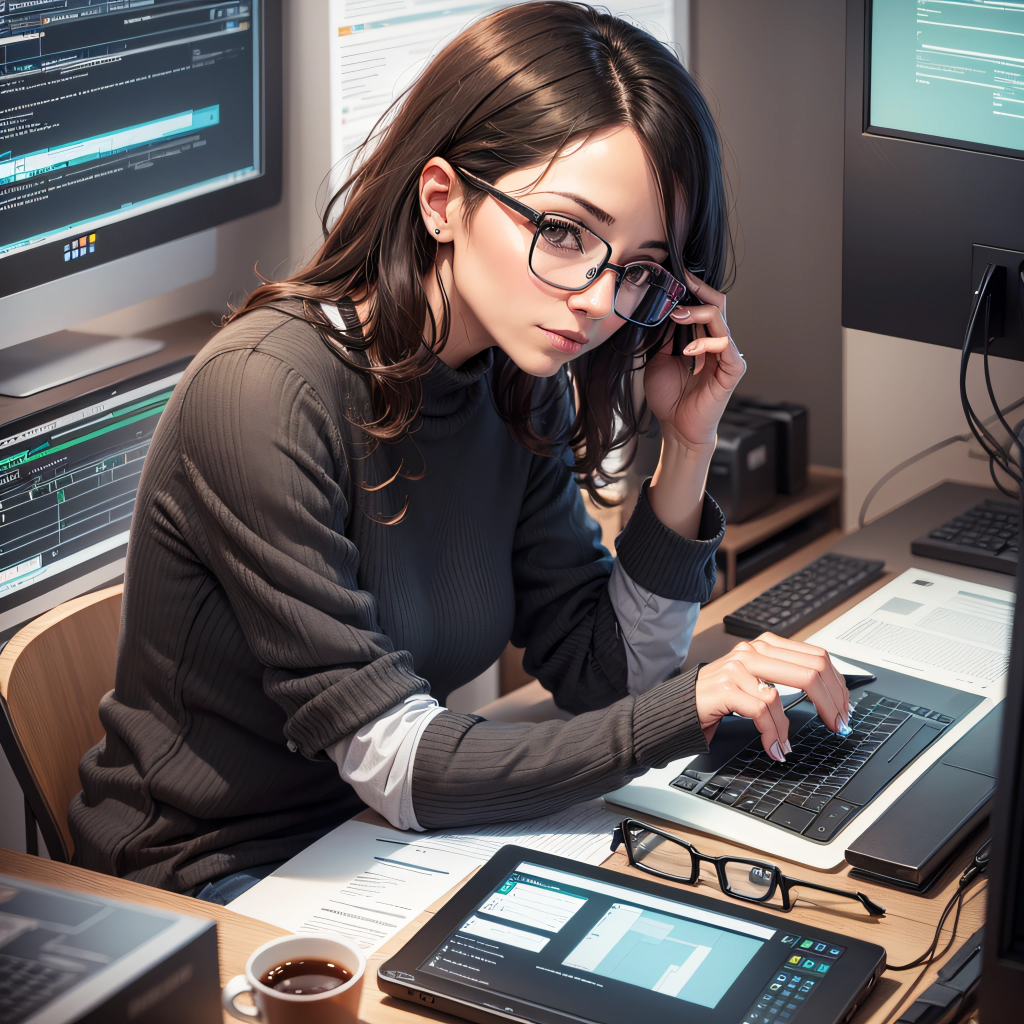
#include <stdio.h>
// Function to draw a horizontal line
void drawLine(int length) {
for(int i = 0; i < length; i++) {
printf("*");
}
printf("\n");
}
int main() {
// Calling the drawLine function
drawLine(10); // Draws a line of 10 asterisks
return 0;
}
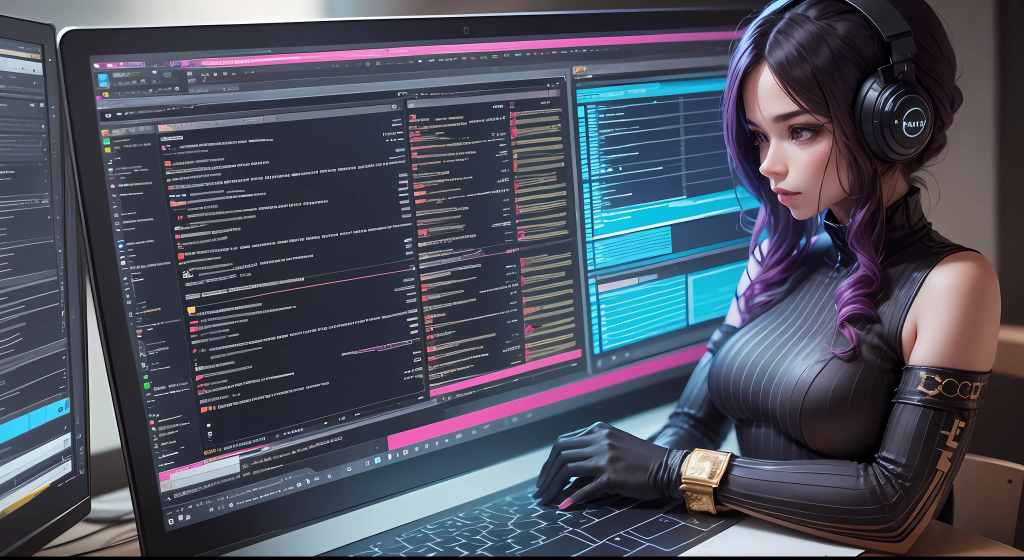
#include <stdio.h>
// Function declarations
void showMenu();
int add(int a, int b);
int subtract(int a, int b);
int multiply(int a, int b);
float divide(int a, int b);
void processChoice(int choice);
int main() {
int choice;
do {
showMenu(); // Display menu
printf("Enter your choice (1-6): ");
scanf("%d", &choice);
if (choice >= 1 && choice <= 5) {
processChoice(choice); // Process the chosen operation
} else if (choice == 6) {
printf("Exiting program...\n");
} else {
printf("Invalid choice, please enter a number between 1 and 6.\n");
}
} while (choice != 6); // Continue until the user chooses to exit
return 0;
}
// Function definitions
void showMenu() {
printf("Menu:\n");
printf("1. Add\n");
printf("2. Subtract\n");
printf("3. Multiply\n");
printf("4. Divide\n");
printf("5. Print Hello\n");
printf("6. Exit\n");
}
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
int multiply(int a, int b) {
return a * b;
}
float divide(int a, int b) {
if (b != 0) {
return (float)a / b;
} else {
printf("Error: Division by zero.\n");
return 0;
}
}
void processChoice(int choice) {
int a, b;
if (choice >= 1 && choice <= 4) {
printf("Enter two numbers: ");
scanf("%d %d", &a, &b);
}
switch (choice) {
case 1:
printf("Result: %d\n", add(a, b));
break;
case 2:
printf("Result: %d\n", subtract(a, b));
break;
case 3:
printf("Result: %d\n", multiply(a, b));
break;
case 4:
printf("Result: %.2f\n", divide(a, b));
break;
case 5:
printf("Hello\n");
break;
default:
// This case should never be reached because of the input validation
printf("Error: Invalid operation.\n");
}
}
Explanation:
- The
showMenu
function displays the options available to the user and does not return any value (void
type). - The
add
,subtract
,multiply
, anddivide
functions perform basic arithmetic operations and return the result. Thedivide
function returns afloat
to accommodate division results, and it handles division by zero. - The
processChoice
function takes the user’s choice, prompts for input if required, performs the chosen operation by calling the appropriate function, and displays the result. This function is also of typevoid
as it does not return any value. - The
main
function displays the menu in a loop, reads the user’s choice, and callsprocessChoice
to handle it, until the user chooses to exit the program by entering6
.
s
#include <stdio.h>
// Function declarations
void showMenu();
double kilometersToMiles(double kilometers);
double milesToKilometers(double miles);
double inchesToCentimeters(double inches);
double metersToKilometers(double meters);
double litersToKilograms(double liters); // This conversion depends on the substance. Assuming water for simplicity.
void processChoice(int choice);
int main() {
int choice;
do {
showMenu(); // Display menu
printf("Enter your choice (1-6): ");
scanf("%d", &choice);
if (choice >= 1 && choice <= 5) {
processChoice(choice); // Process the chosen operation
} else if (choice == 6) {
printf("Exiting program...\n");
} else {
printf("Invalid choice, please enter a number between 1 and 6.\n");
}
} while (choice != 6); // Continue until the user chooses to exit
return 0;
}
// Function to show the conversion menu
void showMenu() {
printf("\nConversion Menu:\n");
printf("1. Kilometers to Miles\n");
printf("2. Miles to Kilometers\n");
printf("3. Inches to Centimeters\n");
printf("4. Meters to Kilometers\n");
printf("5. Liters to Kilograms (water)\n");
printf("6. Exit\n");
}
// Conversion functions
double kilometersToMiles(double kilometers) {
return kilometers * 0.621371;
}
double milesToKilometers(double miles) {
return miles / 0.621371;
}
double inchesToCentimeters(double inches) {
return inches * 2.54;
}
double metersToKilometers(double meters) {
return meters / 1000.0;
}
// Assuming the conversion for water: 1 liter of water is approximately 1 kilogram.
double litersToKilograms(double liters) {
return liters; // For substances other than water, this conversion would be different.
}
// Function to process user choice
void processChoice(int choice) {
double input, result;
switch (choice) {
case 1:
printf("Enter distance in Kilometers: ");
scanf("%lf", &input);
result = kilometersToMiles(input);
printf("%.2f Kilometers = %.2f Miles\n", input, result);
break;
case 2:
printf("Enter distance in Miles: ");
scanf("%lf", &input);
result = milesToKilometers(input);
printf("%.2f Miles = %.2f Kilometers\n", input, result);
break;
case 3:
printf("Enter length in Inches: ");
scanf("%lf", &input);
result = inchesToCentimeters(input);
printf("%.2f Inches = %.2f Centimeters\n", input, result);
break;
case 4:
printf("Enter length in Meters: ");
scanf("%lf", &input);
result = metersToKilometers(input);
printf("%.2f Meters = %.2f Kilometers\n", input, result);
break;
case 5:
printf("Enter volume in Liters: ");
scanf("%lf", &input);
result = litersToKilograms(input);
printf("%.2f Liters of water = %.2f Kilograms\n", input, result);
break;
case 6:
printf("Exiting program...\n");
break;
default:
printf("Invalid choice, please enter a number between 1 and 6.\n");
}
}
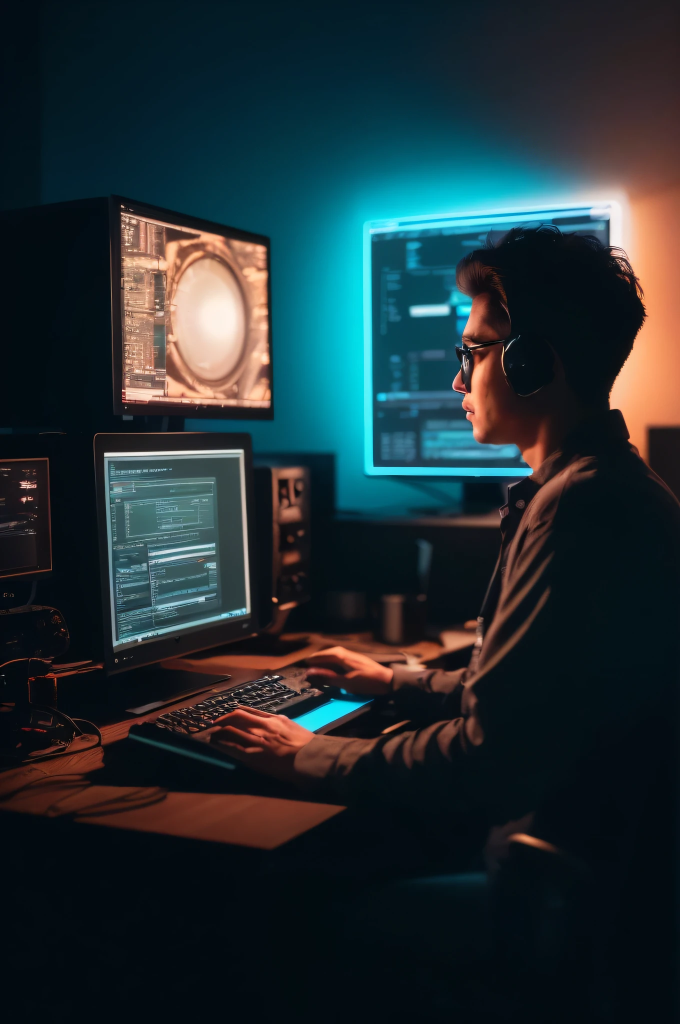
Notes:
- This program assumes the conversion from liters to kilograms is for water, where 1 liter of water is approximately equal to 1 kilogram. For other substances, the density would need to be considered for an accurate conversion.
- The
showMenu
function displays the available conversions to the user. - The
processChoice
function handles user input and output based on the selected conversion operation. - Each conversion function takes an input value, performs the conversion, and returns the result.