Another popular part of websites is menu. Basically, it’s a list of items which are often just simple links pointing to other places on the site. Let’s implement it! We will start with the following HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Menu</title>
<link rel="stylesheet" href="main.css" media="screen">
</head>
<body>
<nav>
<ul>
<li>
<a href="index.html">Home</a>
</li>
<li>
<a href="training.html">Training</a>
</li>
<li>
<a href="conferences.html">Conferences</a>
</li>
<li>
<a href="about.html">About us</a>
</li>
</ul>
</nav>
</body>
</html>
Our menu will consist of four items:
- Home
- Training
- Conferences
- About us
We want it to look something like this:
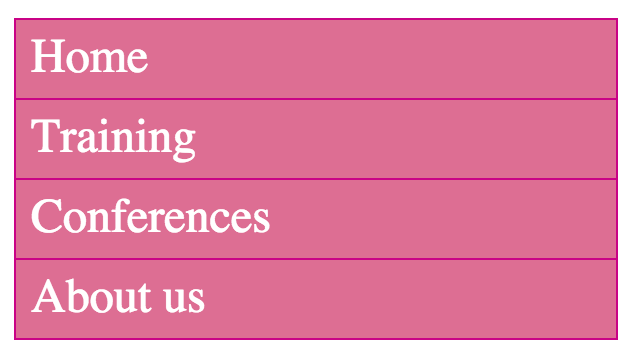
You might notice that under the <body> tag, we’ve added the new tags <nav>, <ul>, and <li>.
<nav> is used for specifying all kinds of navigation functions on websites that contain links to internal or external information. So putting <nav> into the code says “everything inside <nav> will be used to navigate around the website.”
Within <nav>, we place the <ul> tag followed by several <li> tags. The tag <ul> represents an “unordered list” (like a bullet list) and the <li> tags represent each individual component of that list (single bullet). When creating websites, it’s common that an unordered list will be the most reasonable choice when it comes to mapping menu pages. In fact, the menu is kind of a list of links that has been created without a predetermined rule as to the order of its elements.
With just the code above that is still unfinished, our list should be displayed as follows:
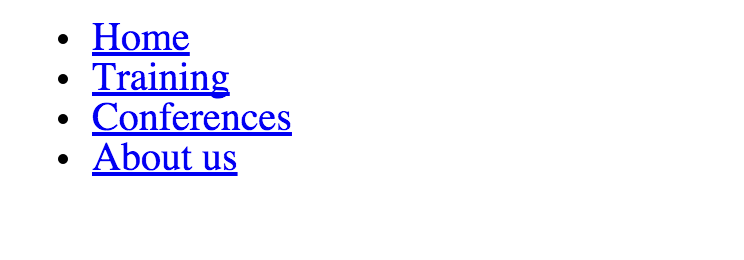
The next tag is then <ul>, which begins the unordered list. We want our list to be displayed slightly differently than the default. The most important thing is to have a new background:
nav ul {
background-color: PaleVioletRed;
}
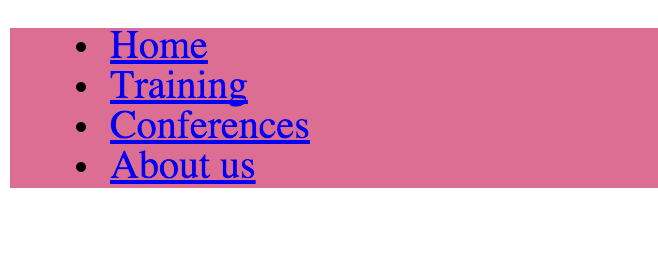
nav ul {
background-color: PaleVioletRed;
list-style: none;
}
It looks much better:
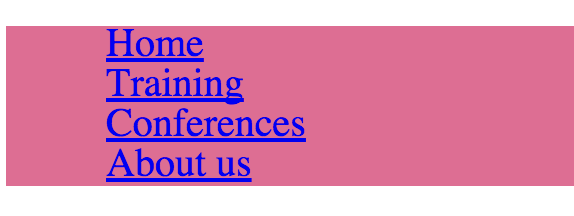
nav ul {
background-color: PaleVioletRed;
list-style: none;
padding: 0;
}
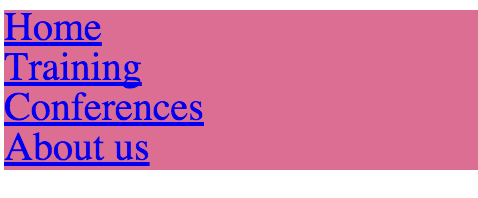
nav ul {
background-color: PaleVioletRed;
list-style: none;
padding: 0;
width: 200px;
border: 1px solid MediumVioletRed;
}
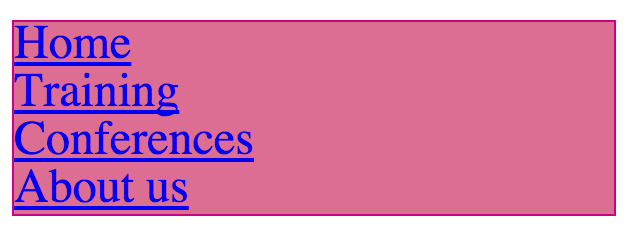
nav ul li {
border-bottom: 1px solid MediumVioletRed;
}
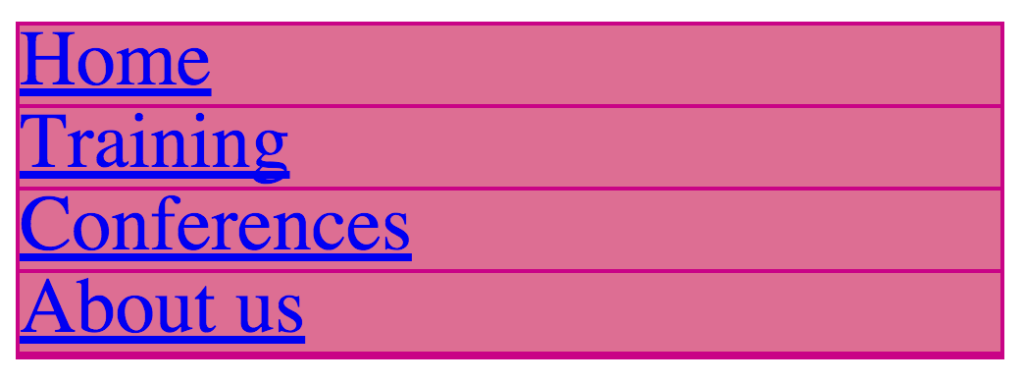
nav ul li { padding: 5px; }
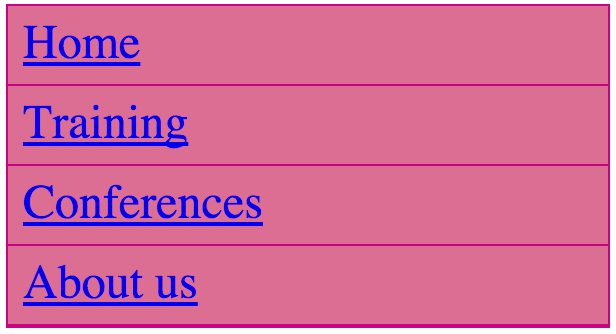
nav ul {
background-color: PaleVioletRed;
list-style: none;
padding: 0;
width: 200px;
border: 1px solid MediumVioletRed;
}
list-style: none;
nav ul li:last-child {
border-bottom: none;
}
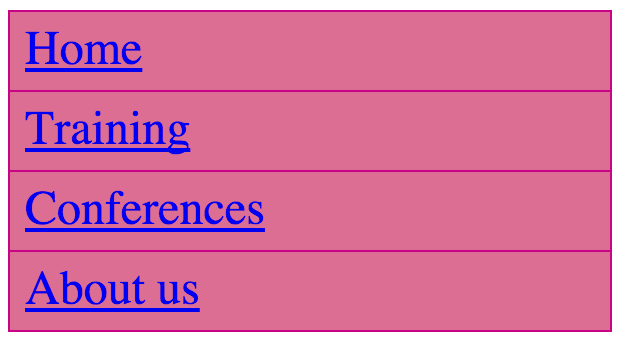
<a href="url">Text entered here will take you to the specified web address</a>
<a href="training.html">Training</a>
nav ul li a {}
nav ul li a {
color: white;
}
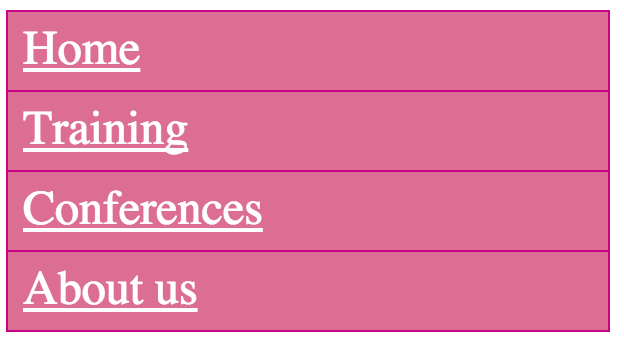
nav ul li a {
color: white;
text-decoration: none;
}
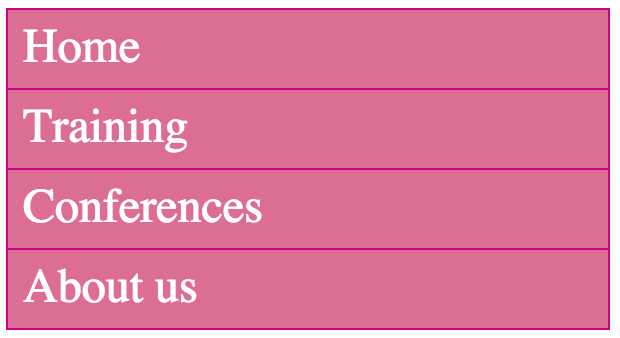
nav ul li a:hover {
text-decoration: underline;
}
In summary, the final CSS code should look like this:
nav ul {
background-color: PaleVioletRed;
list-style: none;
padding: 0;
width: 200px;
border: 1px solid MediumVioletRed;
}
nav ul li {
border-bottom: 1px solid MediumVioletRed;
padding: 5px;
}
nav ul li:last-child {
border-bottom: 0;
}
nav ul li a {
color: white;
text-decoration: none;
}
nav ul li a:hover {
text-decoration: underline;
}