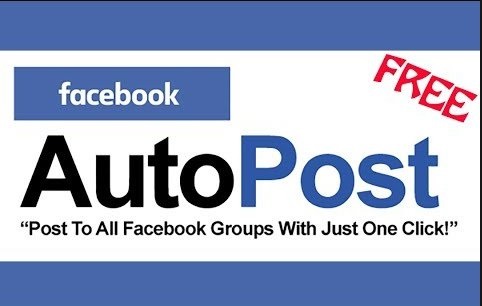
To create a WordPress plugin that automatically adds the necessary Open Graph meta tags
when a post is shared on Facebook, you can follow these steps:
Step 1: Create the Plugin File
- Go to your WordPress installation directory.
- Navigate to
wp-content/plugins/
. - Create a new directory for your plugin, e.g.,
og-meta-tags
. - Inside this directory, create a file named
og-meta-tags.php
.
Step 2: Add Plugin Information and Basic Setup
Open the og-meta-tags.php
file and add the following code:
Here’s the complete og-meta-tags.php
file with the plugin information, settings page, and basic setup included:
<?php
/**
* Plugin Name: OG Meta Tags for Facebook Sharing
* Description: Automatically adds Open Graph meta tags to WordPress posts for better sharing on Facebook. Includes settings for Facebook App ID and Secret.
* Version: 1.0
* Author: Professor Web Developer
*/
// Add a settings menu
function og_meta_tags_menu() {
add_options_page(
'OG Meta Tags Settings',
'OG Meta Tags',
'manage_options',
'og-meta-tags-settings',
'og_meta_tags_settings_page'
);
}
add_action('admin_menu', 'og_meta_tags_menu');
// Display the settings page
function og_meta_tags_settings_page() {
?>
<div class="wrap">
<h1>OG Meta Tags Settings</h1>
<form method="post" action="options.php">
<?php
settings_fields('og_meta_tags_settings');
do_settings_sections('og-meta-tags-settings');
submit_button();
?>
</form>
</div>
<?php
}
// Register settings
function og_meta_tags_settings_init() {
register_setting('og_meta_tags_settings', 'og_meta_tags_app_id');
register_setting('og_meta_tags_settings', 'og_meta_tags_app_secret');
add_settings_section(
'og_meta_tags_section',
'Facebook App Settings',
'og_meta_tags_section_callback',
'og-meta-tags-settings'
);
add_settings_field(
'og_meta_tags_app_id',
'Facebook App ID',
'og_meta_tags_app_id_callback',
'og-meta-tags-settings',
'og_meta_tags_section'
);
add_settings_field(
'og_meta_tags_app_secret',
'Facebook App Secret',
'og_meta_tags_app_secret_callback',
'og-meta-tags-settings',
'og_meta_tags_section'
);
}
add_action('admin_init', 'og_meta_tags_settings_init');
function og_meta_tags_section_callback() {
echo 'Enter your Facebook App credentials:';
}
function og_meta_tags_app_id_callback() {
$app_id = get_option('og_meta_tags_app_id');
echo "<input type='text' name='og_meta_tags_app_id' value='" . esc_attr($app_id) . "' />";
}
function og_meta_tags_app_secret_callback() {
$app_secret = get_option('og_meta_tags_app_secret');
echo "<input type='text' name='og_meta_tags_app_secret' value='" . esc_attr($app_secret) . "' />";
}
// Retrieve Facebook App credentials
function get_facebook_app_credentials() {
$app_id = get_option('og_meta_tags_app_id');
$app_secret = get_option('og_meta_tags_app_secret');
return [
'app_id' => $app_id,
'app_secret' => $app_secret,
];
}
// Add Open Graph meta tags to the head section of each post
function add_og_meta_tags() {
if (is_single()) {
global $post;
$post_thumbnail = get_the_post_thumbnail_url($post->ID, 'full');
$post_description = strip_tags($post->post_excerpt);
if (empty($post_description)) {
$post_description = wp_trim_words($post->post_content, 30, '...');
}
?>
<meta property="og:type" content="article" />
<meta property="og:title" content="<?php echo esc_attr(get_the_title()); ?>" />
<meta property="og:description" content="<?php echo esc_attr($post_description); ?>" />
<meta property="og:image" content="<?php echo esc_url($post_thumbnail); ?>" />
<meta property="og:url" content="<?php echo esc_url(get_permalink()); ?>" />
<?php
}
}
add_action('wp_head', 'add_og_meta_tags');
// Use Facebook credentials (Example of usage with SDK)
function post_to_facebook($link, $message) {
$credentials = get_facebook_app_credentials();
// Example usage: Create Facebook instance using the credentials
// Replace '{access-token}' with the actual access token when using the SDK
$fb = new Facebook\Facebook([
'app_id' => $credentials['app_id'],
'app_secret' => $credentials['app_secret'],
'default_graph_version' => 'v10.0',
]);
try {
$response = $fb->post('/me/feed', [
'link' => $link,
'message' => $message,
], '{access-token}');
return $response->getGraphNode();
} catch (Facebook\Exceptions\FacebookResponseException $e) {
echo 'Graph returned an error: ' . $e->getMessage();
exit;
} catch (Facebook\Exceptions\FacebookSDKException $e) {
echo 'Facebook SDK returned an error: ' . $e->getMessage();
exit;
}
}
?>
Step 3: Activate the Plugin
- Go to the WordPress admin dashboard.
- Navigate to
Plugins
>Installed Plugins
. - Find the “OG Meta Tags for Facebook Sharing” plugin and click
Activate
.
Explanation of the Code:
- is_single(): Checks if the current page is a single post.
- get_the_post_thumbnail_url(): Retrieves the URL of the featured image for the post.
- strip_tags(): Removes any HTML tags from the post excerpt.
- wp_trim_words(): Trims the post content to 30 words if there is no excerpt.
- esc_attr() and esc_url(): Ensures that the data is safely outputted in the meta tags.
Customization:
- You can modify the number of words in
wp_trim_words()
if you need a shorter or longer description. - You can customize the
og:type
or any other tags if your website has specific requirements.
Summary:
- Plugin Setup: This plugin adds Open Graph meta tags to each post, which helps ensure that when your posts are shared on Facebook, the correct information (title, description, image, etc.) is displayed.
- Settings Page: The plugin also includes a settings page where you can securely enter your Facebook App ID and App Secret.
- SDK Integration (Optional): The code includes an example of how you might integrate the Facebook SDK to post content directly to Facebook.
This complete file can be saved as og-meta-tags.php
in your WordPress plugin directory, and then you can activate the plugin through the WordPress admin panel.